
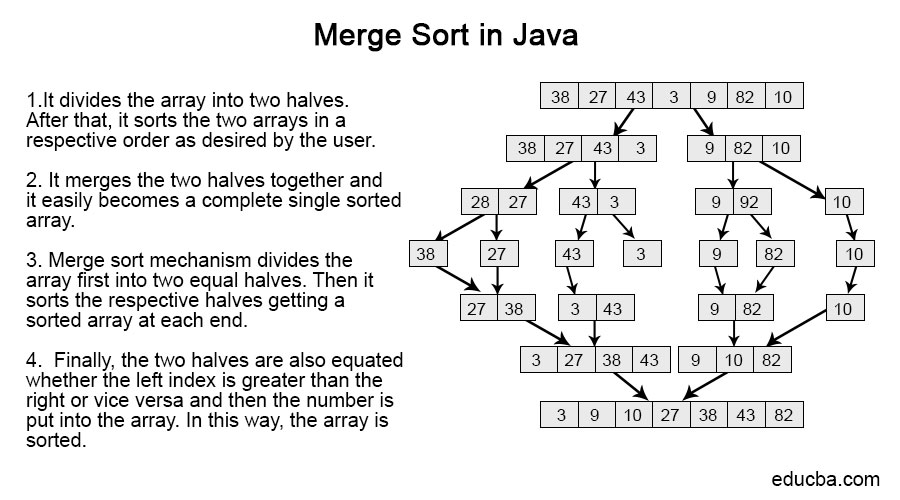
It is because both of them use the divide and conquer approach to sort the elements of the list. Reason: We are using a temporary array to store elements in sorted order. Merge sort is an algorithm that is similar to the quick sort algorithm. If n value is large, it follows divide and conquer approach. Reason: At each step, we divide the whole array, for that logn and we assume n steps are taken to get sorted array, so overall time complexity will be nlogn Merge Sort Algorithm: Merge Sort: One of the best sorting technique. Merge(arr, l, mid, r) // merging sorted halves transfering all elements from temporary to arr // if elements on the right half are still left // storing elements in the temporary array in a sorted manner// Int f = l // index used to transfer elements in temporary array
Merge sorty code#
Step by step instructions on how merging is to be done with the code of merge function.See Complete Playlists. Int j = mid + 1 // starting index of right half of arr Discussed merge sort algorithm with an example. Int i = l // starting index of left half of arr

Void merge(int arr, int l, int mid, int r) > The red number shows an order of the steps in recursion calls > While i Finally will transfer all elements from the temporary array to the original array. > Now, let i = leftmost index of array, j = mid+1 index of array, f = leftmost index of array ( this index will be used to store elements in the original array ) >From the temporary array, we will return back the elements in the original array. This will be used to store elements in sorted order from both the arrays which we divided. > In merge(), we will use a temporary array named temp. MergeSort (a) MergeSort (b) 4) Merge the sorted a and b (using SortedMerge () discussed here) and. FrontBackSplit (head, &a, &b) / a and b are two halves / 3) Sort the two halves a and b. 2) Else divide the linked list into two halves. Where l = leftmost index of array, r = rightmost index of array, mid = middle index of array MergeSort (headRef) 1) If the head is NULL or there is only one element in the Linked List then return. > In mergeSort(), we will divide the array around the middle element by making the recursive call :ġ.mergeSort(arr,l,mid) 2. > We will be creating 2 functions mergeSort() and merge() > We recursively split the array, go from top-down until all sub-arrays size becomes 1. MergeSort() : This function divides the array into 2 parts.It assumes that both part of the array are sorted and merges both of them
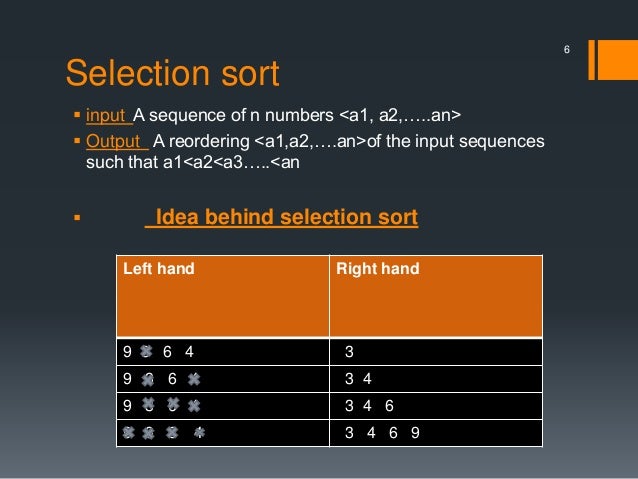
Merge() : This function is used to merge the 2 halves of the array.> Merge Sort is a divide and conquers algorithm, it divides the given array into equal parts and then merges the 2 sorted parts. Merge the two halves sorted in steps 2 and 3.Problem: Given an array of size n, sort the array using Merge Sort.Įxamples: Example 1: Input: N=5, arr=ĭisclaimer: Don’t jump directly to the solution, try it out yourself first. Call the mergeSort method for the second half.Ĥ. Call the mergeSort method for the first half.ģ.
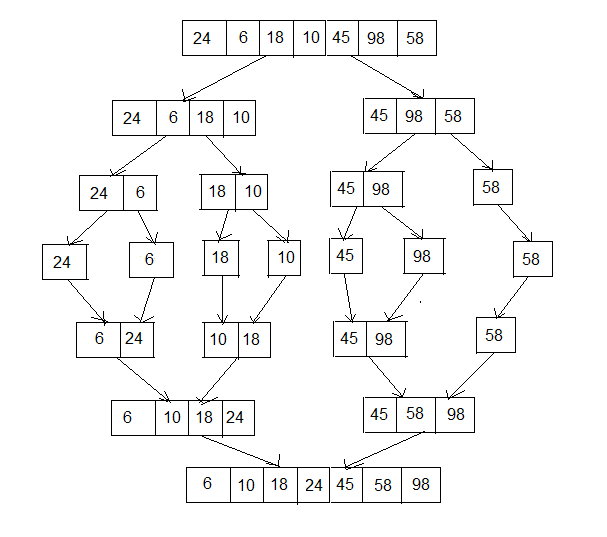
Find the middle to divide the array into two halves m = (g + d) / 2.Ģ. In simple terms merge sort is an sorting algorithm in which it divides the input into equal parts until only two numbers are there for comparisons and then after comparing and odering each parts it merges them all together back to the input. 2) Merge Sort the second half of the list. Here are the steps of Merge Sort: 1) Merge Sort the first half of the list. All of the actual sorting gets done in the Merge method. … Read More Algorithm : mergeSort(tab, g, d)ġ. The answer is that in each call to merge sort, you must run the Merge method on some two parts of the array. The merging process can be performed recursively until there is only one element in the array.ġ00 Multiple Choice Questions In C Programming – Part 1 This collection of 100 Multiple Choice Questions and Answers (MCQs) In C Programming : Quizzes & Practice Tests with Answer focuses on “C Programming”. Once these two arrays are released independently, they are able to produce the sorted array. An array of elements is divided into two smaller sub-arrays. Merge Sort is a recursive algorithm used for merging which is based on the Divide and Conquer technique.
Merge sorty how to#
In this tutorial, we are going to see how to create a Merge Sort program in C.
